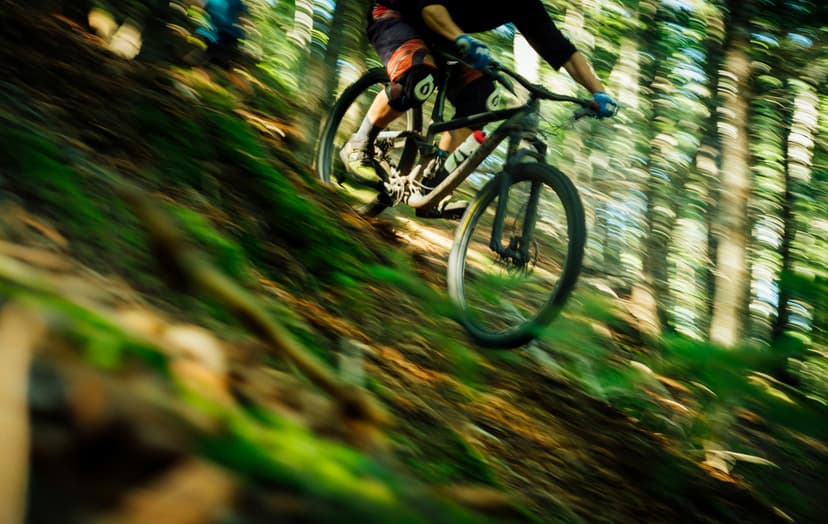
Published on
Nov 10, 2024
Read time
2 min read
Introduction
React is the most popular web framework for building user interfaces. It has proven itself as a powerful choice for building interactive UIs, but as applications grow, performance issues can emerge. A sluggish app can frustrate users, hurt SEO rankings, and potentially lead to lost revenue: back in 2006, a study at Amazon found that every 100ms in added page load time cost them 1% in sales.
Thankfully, React offers a variety of optimization techniques that can help keep your app snappy and responsive. In this article, we’ll explore several techniques to optimise your React applications for speed.
1. Minimize Re-renders with Memoization
// todo - we have been promised a React compiler that makes these optimisations for us, but for now we must still memoise manually
React re-renders components when props or state change, but sometimes, unnecessary re-renders can slow things down, especially in larger applications. Memoization helps prevent these re-renders by caching the output of components and functions so they’re only recalculated when their dependencies change.
Using React.memo React.memo is a higher-order component that can wrap functional components, preventing them from re-rendering when props haven’t changed. Here’s a simple example:
import React from "react";
const ExpensiveComponent = React.memo(({ data }) => {
// This component will only re-render if `data` changes
return <div>{data}</div>;
});
Memoizing Callback Functions with useCallback Sometimes, functions are passed as props, and even though the function itself hasn’t changed, it can still trigger re-renders. To avoid this, use the useCallback hook to memoize these functions:
import React, { useCallback } from "react";
const ParentComponent = () => {
const handleClick = useCallback(() => {
console.log("Button clicked");
}, []);
return <ChildComponent onClick={handleClick} />;
};
Memoization won’t be useful in every scenario, so use it selectively. Generally, it’s beneficial for components that receive complex props or have expensive render processes.
Related articles
You might also enjoy...
Switching to a New Data Structure with Zero Downtime
A Practical Guide to Schema Migrations using TypeScript and MongoDB
7 min read
How to Automate Merge Requests with Node.js and Jira
A quick guide to speed up your MR or PR workflow with a simple Node.js script
7 min read
Automate Your Release Notes with AI
How to save time every week using GitLab, OpenAI, and Node.js
11 min read